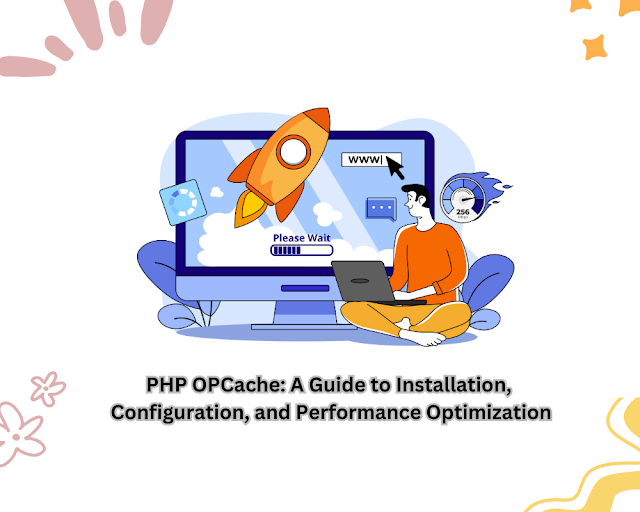
PHP is a powerful and popular server-side scripting language, but like any language, performance can become a concern as your application scales. One of the most effective ways to enhance PHP performance is by using OPCache, a built-in caching mechanism that stores precompiled script bytecode in shared memory. This eliminates the need for PHP to load and parse scripts on every request, leading to faster execution times and reduced CPU load. In this guide, we’ll cover everything you need to know about PHP OPCache, including how to install, configure, enable, and optimize it for your PHP applications.
What is PHP OPCache?
PHP OPCache is a powerful extension that enhances PHP performance by storing precompiled script bytecode in memory, reducing the need for PHP to load and parse scripts on every request. This caching mechanism significantly improves response times and reduces server load, making it a vital tool for high-traffic websites. When a PHP script is executed, it usually goes through the following stages:
Parsing: The script is read and converted into tokens.
Compilation: The tokens are compiled into opcode (operation code), which is a low-level set of instructions that the Zend Engine (PHP's core) can execute.
Execution: The compiled opcode is executed to produce the final output.
Without OPCache, this process happens every time a script is run, which can slow down execution, especially in high-traffic environments. With OPCache enabled, the script is only parsed and compiled once, and the compiled opcode is stored in shared memory. On subsequent requests, the script is served directly from this cache, bypassing the need to parse and compile it again.
Installing and Enabling PHP OPCache
PHP OPCache comes bundled with PHP versions 5.5 and above. While it is included by default, it might not be enabled on your server. Below are the steps to install, enable, and configure PHP OPCache.
Step 1: Check if OPCache is Installed
You can check if OPCache is already installed and enabled by running the following command in your terminal:
php -v
You should see a line that mentions OPcache in the output, which indicates that the extension is available.
Alternatively, you can create a PHP info page to verify:
<?php phpinfo(); ?>
Step 2: Enabling OPCache
If OPCache is not already enabled, you can enable it by adding the line
zend_extension=opcache.so
to your php.ini
file. On
Windows, the line would be zend_extension=php_opcache.dll
. After
adding this line, save the file and proceed to configure OPCache.
Step 3: Basic Configuration
Basic configuration for OPCache can be done by adding specific directives to
your php.ini
file. For example, setting
opcache.memory_consumption=128
allocates 128MB of memory for
OPCache. Adjusting these settings according to your server’s needs can greatly
improve performance.
Step 4: Restart the Web Server
After making changes to the php.ini
file, it is essential to
restart your web server to apply the new settings. For Apache, you can use
sudo systemctl restart apache2
. For Nginx with PHP-FPM, the
commands are sudo systemctl restart php-fpm
and
sudo systemctl restart nginx
.
Configuring OPCache for Optimal Performance
To maximize the performance benefits of OPCache, it's important to fine-tune its configuration. Below are several key settings that can be adjusted to optimize OPCache performance on your server.
Memory Consumption
The opcache.memory_consumption
directive controls how much memory
OPCache can use to store precompiled scripts. Increasing this value allows
OPCache to store more scripts, which is especially beneficial for larger
applications. A typical setting might be
opcache.memory_consumption=128
, but this can be adjusted based on
your server’s resources.
Max Accelerated Files
The opcache.max_accelerated_files
setting determines the maximum
number of files that OPCache can store. Increasing this number is crucial for
applications with a large number of PHP files. A common setting is
opcache.max_accelerated_files=10000
.
Validate Timestamps
By default, OPCache checks the file timestamps every 2 seconds to see if any
scripts have been updated. This behavior can be controlled with the
opcache.revalidate_freq
directive. For a development environment,
you might set opcache.revalidate_freq=0
for immediate validation,
while in production, a higher value is recommended to reduce overhead.
Revalidate Frequency
The opcache.revalidate_freq
setting determines how often OPCache
will check for script changes. A lower value results in more frequent checks,
which can be useful in development environments, but in production, a higher
value like opcache.revalidate_freq=2
can reduce server load.
Preloading (PHP 7.4 and later)
PHP 7.4 introduced script preloading, which allows you to load commonly used
classes and functions into memory at startup. This can be configured with the
opcache.preload
directive in your php.ini
file.
Preloading can significantly reduce the time required to load frequently used
components of your application.
Lockfile Path
The opcache.lockfile_path
directive specifies the path where
OPCache stores its lockfile, used to prevent multiple processes from modifying
the cache simultaneously. Ensuring this path is properly set can prevent cache
corruption and improve stability.
OPCache GUI
An OPCache GUI is a graphical interface that allows you to monitor and manage OPCache usage. Tools like OPCache Status or the PHP OPcache GUI can help you track cache hit rates, memory usage, and other vital statistics, making it easier to optimize your configuration.
Common Troubleshooting Tips
While OPCache can greatly enhance performance, issues may arise that require troubleshooting. Below are some common problems and how to address them.
Cache Invalidation
If your application isn’t reflecting changes immediately, OPCache might be
serving cached content. You can manually invalidate the cache using the
function opcache_reset()
or by setting
opcache.revalidate_freq=0
in your php.ini
file.
Memory Exhaustion
If OPCache runs out of allocated memory, it will stop caching new scripts. You
can resolve this by increasing the
opcache.memory_consumption
value in your
php.ini
file. Monitoring memory usage with an OPCache GUI can
help you determine the appropriate amount of memory to allocate.
Cache Corruption
In rare cases, OPCache might serve corrupted cached files, causing unexpected
behavior in your application. To address this, try resetting the cache with
opcache_reset()
or disabling OPCache temporarily to troubleshoot.
Compatibility Issues
Some PHP applications might experience compatibility issues with OPCache,
especially if they rely on dynamic code generation. In such cases, consider
adjusting OPCache settings like opcache.validate_timestamps
or
temporarily disabling OPCache while debugging.
Conclusion
PHP OPCache is a powerful tool for improving the performance of your PHP applications by caching compiled script bytecode. By properly installing, enabling, and configuring OPCache, you can significantly reduce server load and improve response times, especially in high-traffic environments. Understanding its configuration options and being aware of common issues will help you get the most out of OPCache.
Post a Comment