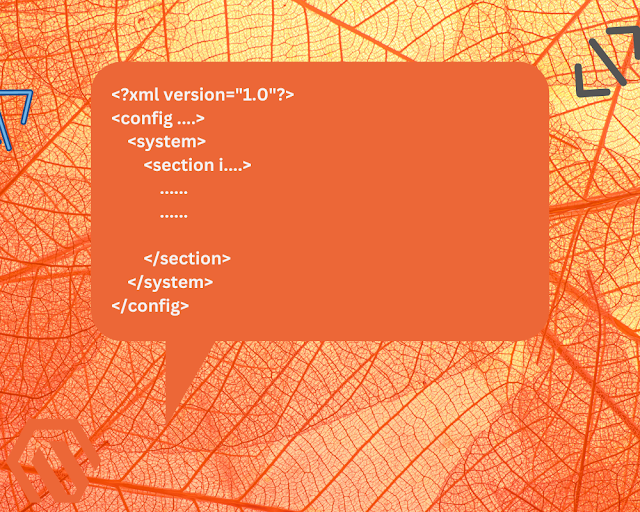
Magento 2 offers a flexible and customizable way to create system configuration fields, allowing us to manage various settings from the admin panel.
Create the Module
Before adding fields to the system configuration, you need to create a custom module. Here's how you can do that.
- Create the module directory:
app/code/Vendor/ModuleName
- Create module.xml inside
app/code/Vendor/ModuleName/etc
:<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd"> <module name="Vendor_ModuleName" setup_version="1.0.0"/> </config>
- Create registration.php in the root of your module:
<?php \Magento\Framework\Component\ComponentRegistrar::register( \Magento\Framework\Component\ComponentRegistrar::MODULE, 'Vendor_ModuleName', __DIR__ );
- Enable the module:
After the module files are created, run the following command to enable it:
php bin/magento setup:upgrade
Now, your module is ready for adding system configuration fields.
Create System Configuration File
1. Create system.xml in app/code/Vendor/ModuleName/etc/adminhtml
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Config/etc/system_file.xsd">
<system>
<section id="custom_section" translate="label" sortOrder="10" showInDefault="1" showInWebsite="1" showInStore="1">
<label>Custom Settings</label>
<tab>general</tab>
<resource>Vendor_ModuleName::config</resource>
<group id="general_settings" translate="label" type="text" sortOrder="10" showInDefault="1" showInWebsite="1" showInStore="1">
<label>General Settings</label>
<field id="custom_field_one" translate="label" type="text" sortOrder="10" showInDefault="1" showInWebsite="1" showInStore="1">
<label>Custom Field One</label>
<comment>This is a custom field.</comment>
</field>
<field id="custom_field_two" translate="label" type="select" sortOrder="20" showInDefault="1" showInWebsite="1" showInStore="1">
<label>Custom Field Two</label>
<source_model>Magento\Config\Model\Config\Source\Yesno</source_model>
<comment>Select Yes or No.</comment>
</field>
<field id="custom_field_three" translate="label" type="textarea" sortOrder="30" showInDefault="1" showInWebsite="1" showInStore="1">
<label>Custom Field Three</label>
<comment>Enter additional information.</comment>
</field>
</group>
</section>
</system>
</config>
Define Permissions for Configuration
1. Create acl.xml in app/code/Vendor/ModuleName/etc/adminhtml
to define permissions:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Acl/etc/acl.xsd">
<acl>
<resources>
<resource id="Magento_Backend::admin">
<resource id="Vendor_ModuleName::config" title="Custom Configuration" sortOrder="10"/>
</resource>
</resources>
</acl>
Access Configuration Values in Code
To use the values set in your system configuration, inject \Magento\Framework\App\Config\ScopeConfigInterface
into your class. Here’s how to retrieve the custom configuration values:
<?php
namespace Vendor\ModuleName\Helper;
use Magento\Framework\App\Helper\AbstractHelper;
use Magento\Store\Model\ScopeInterface;
class Data extends AbstractHelper
{
const XML_PATH_CUSTOM_FIELD_ONE = 'custom_section/general_settings/custom_field_one';
const XML_PATH_CUSTOM_FIELD_TWO = 'custom_section/general_settings/custom_field_two';
public function getCustomFieldOne()
{
return $this->scopeConfig->getValue(self::XML_PATH_CUSTOM_FIELD_ONE, ScopeInterface::SCOPE_STORE);
}
public function getCustomFieldTwo()
{
return $this->scopeConfig->getValue(self::XML_PATH_CUSTOM_FIELD_TWO, ScopeInterface::SCOPE_STORE);
}
}
Once you've added the fields to system.xml
, cleared your cache, and reloaded the admin panel, you should see the new configuration section with your custom fields under Stores > Configuration > General > Custom Settings.
Post a Comment